Vuex
Vuex 是实现组件全局状态(数据)管理的一种机制,可以方便的实现组件之间数据的共享。
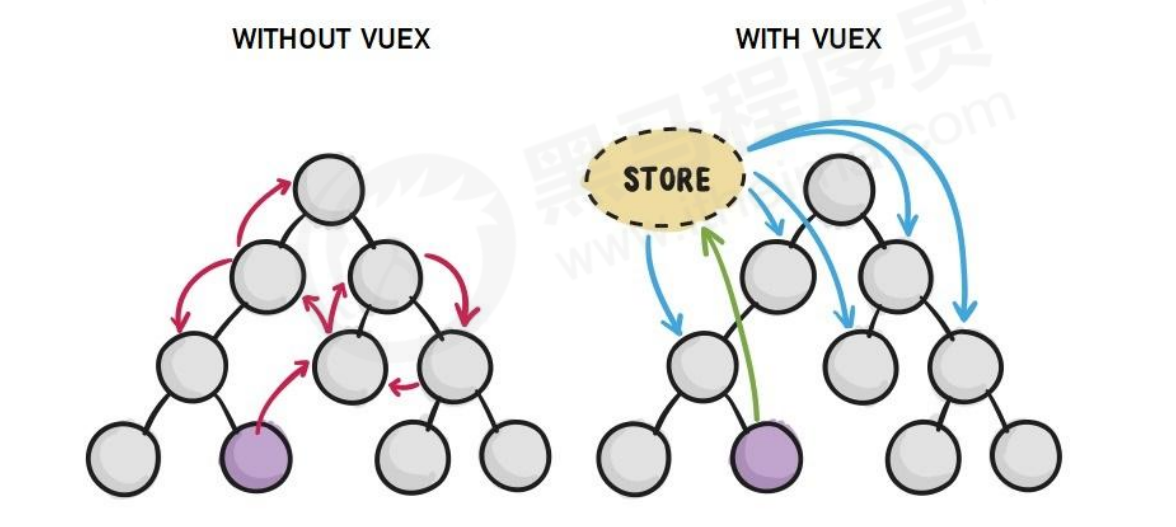
① 能够在 vuex 中集中管理共享的数据,易于开发和后期维护
② 能够高效地实现组件之间的数据共享,提高开发效率
③ 存储在 vuex 中的数据都是响应式的,能够实时保持数据与页面的同步
Vuex基本使用
- 安装 vuex 依赖包
npm install vuex --save
- 导入 vuex 包
1 2 3 4
| import Vuex from 'vuex'
Vue.use(Vuex)
|
- 创建 store 对象
1 2 3 4 5 6 7 8
| const store = new Vuex.Store({
// state 中存放的就是全局共享的数据
state: { count: 0 }
})
|
- 将 store 对象挂载到 vue 实例中
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| new Vue({
el: '#app',
render: h => h(app),
router,
store
})
|
核心概念
state
State 提供唯一的公共数据源,所有共享的数据都要统一放到 Store 的 State 中进行存储。
1 2 3 4
| const store = new Vuex.Store({ state: { count: 0 } })
|
组件访问 State 中数据的第一种方式:
this.$store.state.全局数据名称
组件访问 State 中数据的第二种方式:
1 2 3 4 5 6
| import { mapState } from 'vuex'
computed: { ...mapState(['count']) }
|
mutation
Mutation 用于变更 Store中 的数据。
① 只能通过 mutation 变更 Store 数据,不可以直接操作 Store 中的数据。
② 通过这种方式虽然操作起来稍微繁琐一些,但是可以集中监控所有数据的变化。
(不允许组件直接去修改store中的数据)
1 2 3 4 5 6 7 8 9 10 11 12
| const store = new Vuex.Store({ state: { count: 0 }, mutations: { add(state) {
state.count++ } } })
|
1 2 3 4 5 6 7
| methods: { handle1() { this.$store.commit('add') } }
|
可以在触发mutations时传递参数
1 2 3 4 5 6 7 8 9 10 11 12
| const store = new Vuex.Store({ state: { count: 0 }, mutations: { addN(state, step) {
state.count += step } } })
|
1 2 3 4 5 6 7 8
| methods: { handle2() {
this.$store.commit('addN', 3) } }
|
触发 mutations 的第二种方式
1 2
| import { mapMutations } from 'vuex'
|
通过刚才导入的 mapMutations 函数,将需要的 mutations 函数,映射为当前组件的 methods 方法:
1 2 3 4 5
| methods: { ...mapMutations(['add', 'addN']) } 之后就可以调用this.add(),this.addN()方法
|
mutations无法执行异步操作,如果mutations中定义延时函数去改变state,页面上会有变化但是调试工具中没有变化
action
Action 用于处理异步任务。
如果通过异步操作变更数据,必须通过 Action,而不能使用 Mutation,但是在 Action 中还是要通过触发
Mutation 的方式间接变更数据。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| const store = new Vuex.Store({
mutations: { addN(state, step) { state.count += step } }, actions: { addNAsync(context, step) { setTimeout(() => { context.commit('addN', step) }, 1000) } } })
|
1 2 3 4 5 6 7 8 9 10
| methods: { handle() { this.$store.dispatch('addNAsync', 5) } }
|
触发 actions 的第二种方式(和mutation类似)
1 2 3 4 5 6
| import { mapActions } from 'vuex'
methods: { ...mapActions(['addASync', 'addNASync']) }
|
Getter
Getter 用于对 Store 中的数据进行加工处理形成新的数据。
① Getter 可以对 Store 中已有的数据加工处理之后形成新的数据,类似 Vue 的计算属性。
② Store 中数据发生变化,Getter 的数据也会跟着变化
1 2 3 4 5 6 7 8 9 10 11
| const store = new Vuex.Store({ state: { count: 0 }, getters: { showNum: state => { return '当前最新的数量是【'+ state.count +'】' } } })
|
使用 getters 的第一种方式:
this.$store.getters.名称
使用 getters 的第二种方式:
1 2 3 4
| import { mapGetters } from 'vuex' computed: { ...mapGetters(['showNum']) }
|