JS学习笔记11
==正则表达式==
使用场景
验证表单
过滤敏感词(替换)
从字符串中获取想要的特定部分(提取)
语法
- 定义规则
其中/ /
是正则表达式字面量
- 是否匹配
1 2 3 4 5
| test() 方法用来查看是否匹配
regObj.test(被检查的字符串) regObj为定义的规则
|
如果正则表达式与指定的字符串匹配 ,返回true
,否则false
元字符
是一项具有特殊意义的字符,可以极大提高了灵活性和强大的匹配功能
分类
边界符 |
说明 |
^ |
表示匹配行首的文本,以谁开头 |
$ |
表示匹配行末的文本,以谁结尾 |
如果 ^ 和 $ 在一起,表示必须是精确匹配
用来设定某个模式出现的次数
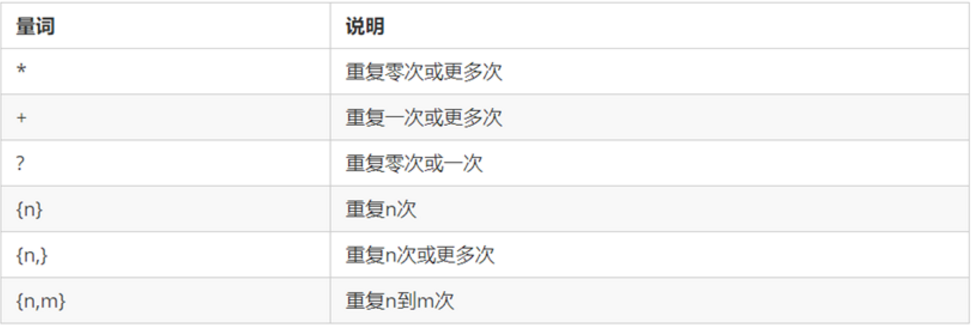
注意: 逗号左右两侧千万不要出现空格
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
| <body> <script> const reg1 = /^w*$/ console.log(reg1.test('')) console.log(reg1.test('w')) console.log(reg1.test('ww')) console.log('-----------------------')
const reg2 = /^w+$/ console.log(reg2.test('')) console.log(reg2.test('w')) console.log(reg2.test('ww')) console.log('-----------------------')
const reg3 = /^w?$/ console.log(reg3.test('')) console.log(reg3.test('w')) console.log(reg3.test('ww')) console.log('-----------------------')
const reg4 = /^w{3}$/ console.log(reg4.test('')) console.log(reg4.test('w')) console.log(reg4.test('ww')) console.log(reg4.test('www')) console.log(reg4.test('wwww')) console.log('-----------------------')
const reg5 = /^w{2,}$/ console.log(reg5.test('')) console.log(reg5.test('w')) console.log(reg5.test('ww')) console.log(reg5.test('www')) console.log('-----------------------')
const reg6 = /^w{2,4}$/ console.log(reg6.test('w')) console.log(reg6.test('ww')) console.log(reg6.test('www')) console.log(reg6.test('wwww')) console.log(reg6.test('wwwww'))
</script>
|
[]匹配字符集合(==只选一个!==)
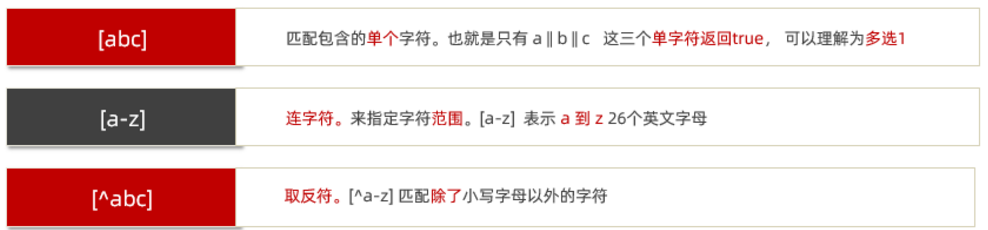
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
| <body> <script> const reg1 = /^[abc]$/ console.log(reg1.test('a')) console.log(reg1.test('b')) console.log(reg1.test('c')) console.log(reg1.test('d')) console.log(reg1.test('ab'))
const reg2 = /^[a-z]$/ console.log(reg2.test('a')) console.log(reg2.test('p')) console.log(reg2.test('0')) console.log(reg2.test('A')) const reg3 = /^[a-zA-Z0-9]$/ console.log(reg3.test('B')) console.log(reg3.test('b')) console.log(reg3.test(9)) console.log(reg3.test(','))
const reg4 = /^[a-zA-Z0-9_]{6,16}$/ console.log(reg4.test('abcd1')) console.log(reg4.test('abcd12')) console.log(reg4.test('ABcd12')) console.log(reg4.test('ABcd12_'))
const reg5 = /^[^a-z]$/ console.log(reg5.test('a')) console.log(reg5.test('A')) console.log(reg5.test(8))
</script> </body>
|
字符类(等于上面模式的更一步简化)
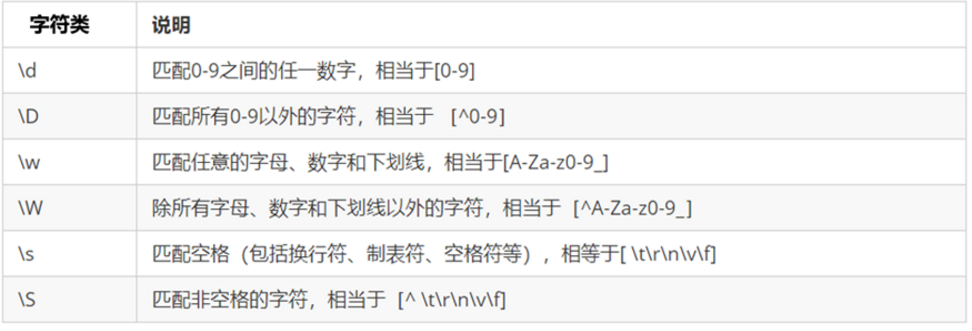
修饰符和替换
1 2 3 4 5 6 7 8 9
| <body> <script> const str = '欢迎大家学习前端,相信大家一定能学好前端,都成为前端大神' </script> </body>
|
修饰符约束正则执行的某些细节行为,如是否区分大小写、是否支持多行匹配等
- i 是单词 ignore 的缩写,正则匹配时字母不区分大小写
- g 是单词 global 的缩写,匹配所有满足正则表达式的结果
1 2 3 4 5 6 7 8 9 10 11 12 13
| <body> <script> const str = '欢迎大家学习前端,相信大家一定能学好前端,都成为前端大神'
const strEnd = str.replace(/前端/g, 'web') console.log(strEnd) </script> </body>
|